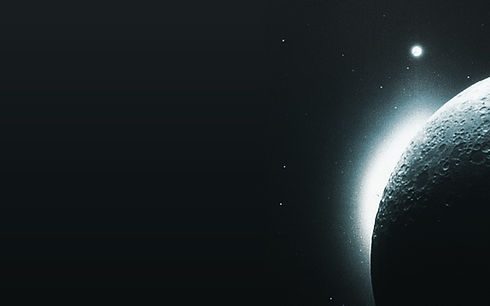
SPACE TRAVEL
My most extensive Computer Science project yet.
This program was created in part with my intro level computer science course, and was the most challenging and rewarding project I have had so far. The objective for this project was to create a menu based program with multiple different problems that require different algorithms to test our knowledge with different algorithms
THE CODE
menu=0
# Main menu function that loops if not given 1,2, or 3 and quits if given 4, and will loop after putting correct input and user wants to try new function
while menu != 4:
print()
print()
print('Main menu:')
print('1. Warp Speed')
print('2. Cost to Launch')
print('3. Time Dilation')
print('4. Quit')
menu = int(input('Choose a function: '))
# Calculates warp factor/ warp speed
if menu == 1:
#assigned variables
warp_factor = -1
speed_of_light = 299792458
warp_exponent = 10/3
#while not statement makes sure warp factor is greater than 0
while not warp_factor >= 0:
warp_factor = int(input('Enter warp factor: '))
if warp_factor >=0:
warp_speed = (warp_factor**(warp_exponent) * speed_of_light)
print()
print('Warp',warp_factor,'engage!')
print ('You are now traveling at',"{0:,.2f}".format(warp_speed), 'meters/second')
# calculates cost to launch and lets you know how much you save whether using space x or ULA
elif menu == 2:
#assinged variables for the while statements, and variables of the given rates for price per kilogram launched
satellite_mass = -1
satellite_cost = -1
spacex_rate = 2720
ula_rate = 14830
spacex_insurance = .30
#validates mass and oost of satellites then calculates
while not satellite_mass > 0:
satellite_mass = float(input('Enter satellite mass in kilograms: '))
if satellite_mass > 0:
while not satellite_cost > 0:
satellite_cost = float(input('Enter satelllite cost in dollars: '))
if satellite_cost > 0:
# calculates SpaceX insurance and adds it to total and calculates total for ULA
spacex_insurance *= satellite_cost
spacex_total = spacex_rate * satellite_mass + spacex_insurance
ula_total = ula_rate * satellite_mass
#Next few lines tell you how much you saved using the cheaper option
if spacex_total < ula_total:
spacex_saved = ula_total - spacex_total
print()
print('SpaceX will save you',"{0:,.2f}".format(spacex_saved),'on this launch')
elif ula_total < spacex_total:
ula_saved = spacex_total - ula_total
print()
print('United Launch Alliance will save you',"{0:,.2f}".format(ula_saved),'on this launch')
else:
print('SpaceX and ULA will charge the same') #Function if both produce the same output
# Calculates time on earth and time dilation from space
elif menu == 3:
# Assinged variables for while statements and for math used
user_speed = 0
user_distance = 0
year = 365
light_year = 9460730472580800
speed_of_light = 299792458
#while statements validate speed and distance
while not (user_speed >0 and user_speed < 1):
user_speed = float(input('Speed of space ship, expressed as a fraction of the speed of light: '))
while not user_distance >0:
user_distance = float(input('Enter travel distance in light years: '))
#below statements till elif of earth calculate earth observer time
earth_time = user_distance / user_speed
earth_time *= year
# if less than 1 year just prints total days
if earth_time <365:
print()
print('An observer on Earth ages',"{0:,.0f}".format(earth_time),'days during the trip')
print()
#if more than a year prints amount of years and total days
elif earth_time>=365:
earth_days = earth_time % 365
earth_years = (earth_time - earth_days) / year
print()
print('An observer on Earth ages',"{0:,.0f}".format(earth_years),'years and',"{0:,.0f}".format(earth_days),'days during the trip')
print()
#calculates time on space aka time dilation
# the math may look weird but it calculates the square root and when multiplying velocity by c it cancels out
# the bottoom c so you can just use speed as a fraction of the speed of light and still get the right answer
# you then multiply the time dilation times total earth days and it gives you the answer
#CALCULATIONS FOR TIME DILATION
import math
time_dilation = (math.sqrt((1 - (user_speed**2)))) * (earth_time)
#if less than 1 years just prints days
if time_dilation <365:
print('A passenger on the ships ages',"{0:,.0f}".format(time_dilation),'days during the trip')
# if more than a year prints out total years and days
elif time_dilation >=365:
space_days = time_dilation % 365
space_years = (time_dilation - space_days) / year
print('A passenger on the ship ages',"{0:,.0f}".format(space_years),'years and',"{0:,.0f}".format(space_days),'days during the trip')
#NOTHING FOR FUNCTION 4 BECAUSE IF USER INPUTS 4 IT SIMPLY QUITS