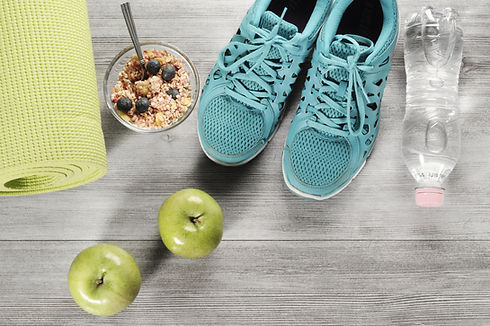
IF IT FITS YOUR MACROS
March 2, 2019
With fitness, comes eating the proper amount of calories and macro nutrients. Macro nutrients work in a way that you can eat any "bad" food as long as it fits within your macro nutrient goal. I created a program that the user is able to input any percentages of fats, carbs, and protein and  input a meal they would like to eat and the program outputs how much more protein, carbs or fat they need to eat to reach their macro nutrient percentage goals.
THE CODE
protein_str = 'protein'
fat_str = 'fat'
carbs_str = 'carbs'
def carbs():
carbs = float(input('Enter total carbs: '))
return carbs
def protein():
protein = float(input('Enter total protein: '))
return protein
def fat():
fat = float(input('Enter total fat: '))
return fat
def total():
net_macros = carbs + protein + fat
return net_macros
def percentages(macro):
percent_macro = int(input("Enter percentage amount of %s (All must add up to 100) : " % macro))
return percent_macro
carbs = carbs()
protein = protein()
fat = fat()
total = total()
prc_carbs = percentages(carbs_str)
prc_protein = percentages(protein_str)
prc_fat = percentages(fat_str)
def ate_prc(macro,total):
ate_prc = (macro/total)*100
return ate_prc
prc_ate_carbs = ate_prc(carbs,total)
prc_ate_protein = ate_prc(protein,total)
prc_ate_fat = ate_prc(fat,total)
def biggest(prc_ate_carbs, prc_ate_protein, prc_ate_fat):
if prc_ate_carbs > prc_ate_protein and prc_ate_carbs > prc_ate_fat:
return prc_ate_carbs
elif prc_ate_protein > prc_ate_fat and prc_ate_protein > prc_ate_carbs:
return prc_ate_protein
else:
return prc_ate_fat
biggest = biggest(prc_ate_carbs, prc_ate_protein, prc_ate_fat)
def must_eat(biggest,total,prc_protein,prc_fat,prc_carbs):
biggest = (biggest / 100) * total
if biggest == protein:
good_total = (100/ prc_protein)* protein
carb_total = good_total * (prc_carbs * .01)
fat_total = good_total * (prc_fat * .01)
print('If you ate',biggest,'amount of protein you need',carb_total,'amount of carbs and ',fat_total,'amount of fat.')
elif biggest == carbs:
good_total = (100/ prc_carbs)* carbs
protein_total = good_total * (prc_protein * .01)
fat_total = good_total * (prc_fat * .01)
print('If you ate',biggest,'amount of carbs you need',protein_total,'amount of protein and ',fat_total,'amount of fat.')
else:
good_total = (100/ prc_fat)* fat
carb_total = good_total * (prc_carbs * .01)
protein_total = good_total * (prc_protein * .01)
print('If you ate',biggest,'amount of fat you need',carb_total,'amount of carbs and ',protein_total,'amount of protein.')
must_eat(biggest, total,prc_protein,prc_fat,prc_carbs)